2024. 6. 14. 23:55ㆍIT/BaekJoon
문제 링크
https://www.acmicpc.net/problem/9850
문제
You are the leader of a crack intelligence unit, and today your team intercepted a set of encrypted messages (”ciphertexts”) from “Kojak”, a well known and much feared terrorist leader. It is thought that these ciphertexts contain instructions to his henchmen on which targets to attack next. One of the ciphertexts reads as follows:
XLMW MW OSNEO. M EQ LIVIFC SVHIVMRK XLEX EPP QC QIR QYWX IEX TITTIVSRM TMDDEW IZIVCHEC. XLMW SVHIV AMPP FI VITIEPIH SRPC YTSR QC VIXMVIQIRX. PSRK PMZI XLI GLMTQYROW!
Your team knows that despite Kojak’s sophistication, he uses a simple Caesar Cipher. In this cipher, letters are shifted by a fixed number of positions P, where P is unknown to you. For example, if letters are shifted by P=2 positions, we get the following “key”:

So, for example, HELLO WORLD is encrypted to JGNNQ YQTNF. Kojak encrypts only upper-case letters.
Your team also knows that the words “CHIPMUNKS” and “LIVE” always appear in Kojak’s messages, and that he uses a different key for each message. You can assume that for each message, there is exactly one key that results in the words “CHIPMUNKS” and “LIVE” to appear in the decrypted message. Your task is to write a program to decrypt all of Kojak’s encrypted messages (not just the one shown above) to produce the corresponding plaintext. The fate of your nation is in your hands!
입력
This is a text file containing a single line of ciphertext consisting of at most 1,000 characters.
출력
The plaintext version of the ciphertext. The plaintext version has exactly the same characters as the ciphertext (including newlines, spaces etc), except that uppercase letters are replaced by their decrypted version. Thus, Kojak’s message above leads to the following output:
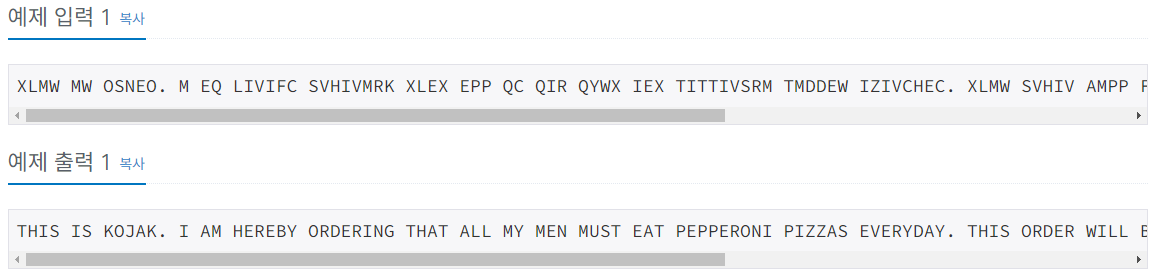
통과한 답안
using System.Text;
namespace _9850
{
internal class Program
{
static void Main(string[] args)
{
string input = Console.ReadLine();
StringBuilder sb = new StringBuilder();
for (int i = 1; i < 26; i++)
{
foreach (char c in input)
{
if (c >= 'A' && c <= 'Z')
{
char alp = (char)(c - i);
if (alp < 'A')
{
alp = (char)(alp + 26);
}
sb.Append(alp);
}
else
{
sb.Append(c);
}
}
string answer = sb.ToString();
if (answer.Contains("CHIPMUNKS") && answer.Contains("LIVE"))
{
Console.WriteLine(answer);
return;
}
else
{
sb.Clear();
}
}
}
}
}
암호화된 문자열이 주어진 경우, 해독한 문자열을 출력하는 문제이다.
문자열을 암호화하는 방법은 시저 암호를 사용하는 것으로
각 문자를 일정한 위치만큼 이동시키는 방식을 사용한다.
또, 올바른 문자열인 것을 확인하기 위해 "CHIPMUNKS"와 "LIVE"가
해독한 문자열에 포함되어야 한다.
이 문제를 해결하기 위해서 주어진 input 값의 모든 문자에 대해서
A와 Z 사이에 있는 문자는 특정 값(1부터 26만큼)만큼 이동한 문자를
그 외의 문자는 입력된 문자를 StringBuilder를 이용하여 문자열을 만들고
이 문자열에 "CHIPMUNKS"와 "LIVE"가 포함되어 있는지 확인하도록 구현하였다.
'IT > BaekJoon' 카테고리의 다른 글
[BAEKJOON] 백준 15667: 2018 연세대학교 프로그래밍 경진대회 (C#) (0) | 2024.06.16 |
---|---|
[BAEKJOON] 백준 11576: Base Conversion (C#) (1) | 2024.06.15 |
[BAEKJOON] 백준 19947: 투자의 귀재 배주형 (C#) (0) | 2024.06.14 |
[BAEKJOON] 백준 6438: Reverse Text (C#) (0) | 2024.06.14 |
[BAEKJOON] 백준 1783: 병든 나이트 (C#) (0) | 2024.06.13 |