2024. 8. 5. 09:14ㆍIT/BaekJoon
문제 링크
https://www.acmicpc.net/problem/14011
문제
"There is probably a place where all waiters have a PhD in, I don't know, some stuff." - Dudu, 2014
Note: This problem is identical to Large PhD Restaurant, but with smaller bounds.
Dudu is hungry. He sat down in a nice Thai restaurant. To his amazement, he realized that he is in the PhD restaurant, a wonderful place where every staff member has a PhD in some stuff.
Even more, each staff member has arranged a challenge related to his or her field of study for Dudu to attempt while he waits for his food. The challenge arranged by staff member i costs Ai to attempt and will award Dudu Biwhen he completes it successfully.
Each challenge can be completed at most once and they can be attempted in any order. Dudu is very smart and can complete any challenge, but he must have enough money to pay Ai before he attempts challenge i. If Dudu starts with M money, determine the maximum amount he can obtain.
입력
Input begins with a line containing two integers N and M, the number of challenges and the amount of money Dudu begins with, respectively.
The following line contains N numbers A1,A2, ..., AN, indicating the costs to attempt each challenge.
Finally, the last line contains the N numbers B1, B2, ..., BN indicating the payoff from each challenge.
- 1 ≤ N ≤ 1000
- 0 ≤ M, Ai, Bi ≤ 106
출력
Output a single integer indicating the maximum amount of money Dudu can obtain.
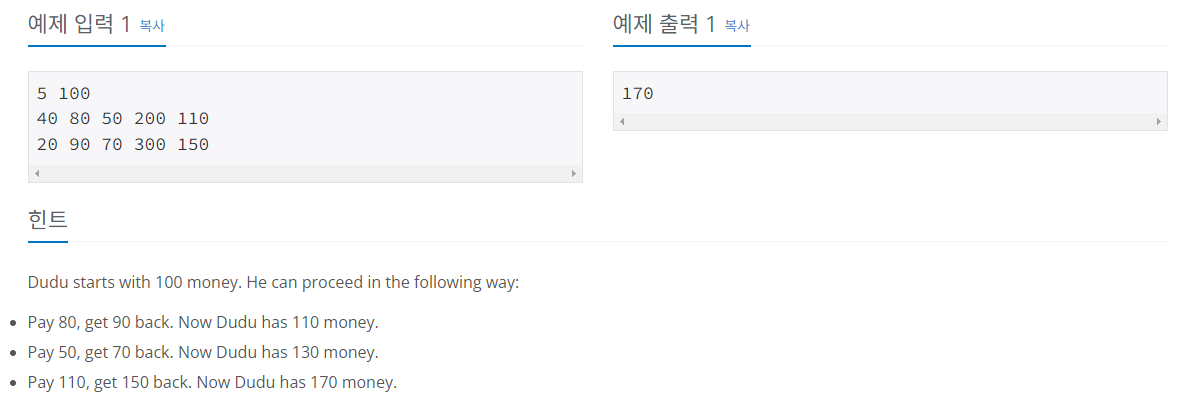
통과한 답안
namespace _14011
{
internal class Program
{
static void Main(string[] args)
{
string[] inputs = Console.ReadLine().Split(' ');
int N = int.Parse(inputs[0]);
int M = int.Parse(inputs[1]);
int[] A = new int[N];
string[] AInputs = Console.ReadLine().Split(' ');
for (int i = 0; i < N; i++)
{
A[i] = int.Parse(AInputs[i]);
}
int[] B = new int[N];
string[] BInputs = Console.ReadLine().Split(' ');
for (int i = 0; i < N; i++)
{
B[i] = int.Parse(BInputs[i]);
}
List<(int cost, int reward)> challenges = new List<(int, int)>();
for (int i = 0; i < N; i++)
{
challenges.Add((A[i], B[i]));
}
challenges.Sort((x, y) => x.cost.CompareTo(y.cost));
int currentMoney = M;
foreach (var challenge in challenges)
{
if (currentMoney >= challenge.cost && challenge.reward > challenge.cost)
{
currentMoney += challenge.reward - challenge.cost;
}
}
Console.WriteLine(currentMoney);
}
}
}
N개의 테스트 수와 시작 자금 M이 주어지고
각각의 테스트를 하기 위한 금액 Ai와 테스트를 통과하여 얻을 수 있는 금액 Bi가 주어진다.
이 경우에 최대로 확보할 수 있는 금액을 구하는 문제로
각각의 입력값을 받은 후에 필요한 금액(cost)과 얻을 수 있는 금액(reward)를
하나의 List로 묶어준 후에 이를 필요한 금액 순으로 정렬하였다.
이후에 각각의 테스트에 대해서 현재 금액이 테스트에 필요한 금액보다 많으며,
테스트의 보상이 필요한 금액보다 많은 경우에 자금에 더하도록 구현하였다.
(여기에서 필요한 금액 순으로 정렬하였기 때문에 보상을 받기 전에는 수행할 수 없다가
보상을 받은 후에 수행할 수 있는 테스트는 존재하지 않는다)
문제 자체는 어렵지 않으나 문제의 오류인지 런타임 에러(Format)이 발생하였다.
int[] A = Console.ReadLine().Split(' ').Select(int.Parse).ToArray();
int[] B = Console.ReadLine().Split(' ').Select(int.Parse).ToArray();
이전에 사용하던 코드였던 위의 코드를 아래의 코드로 수정하여 해결할 수 있었다.
int[] A = new int[N];
string[] AInputs = Console.ReadLine().Split(' ');
for (int i = 0; i < N; i++)
{
A[i] = int.Parse(AInputs[i]);
}
int[] B = new int[N];
string[] BInputs = Console.ReadLine().Split(' ');
for (int i = 0; i < N; i++)
{
B[i] = int.Parse(BInputs[i]);
}
'IT > BaekJoon' 카테고리의 다른 글
[BAEKJOON] 백준 11728: 배열 합치기 (C#) (0) | 2024.08.07 |
---|---|
[BAEKJOON] 백준 10480: Oddities (C#) (0) | 2024.08.07 |
[BAEKJOON] 백준 31846: 문자열 접기 (C#) (0) | 2024.08.05 |
[BAEKJOON] 백준 31775: 글로벌 포닉스 (C#) (0) | 2024.08.03 |
[BAEKJOON] 백준 31495: 그게 무슨 코드니.. (C#) (0) | 2024.08.02 |