2024. 8. 15. 09:28ㆍIT/BaekJoon
문제 링크
https://www.acmicpc.net/problem/16175
문제
General Election is over, now it is time to count the votes! There are N (2 <= N <= 5) candidates and M (1 <= M <= 100) vote regions. Given the number of votes for each candidate from each region, determine which candidate is the winner (one with the most votes).
입력
The first line of input contains an integer T, the number of test cases follow.
Each test case starts with an integer N and M denoting the number of candidate and number of region. The next M lines each contains N integers, v1, v2, ..., vN (0 <= vi <= 1000) the number of votes for candidate i.
출력
For each test case, output in a line the winning candidate. You may safely assume that the winner is unique.
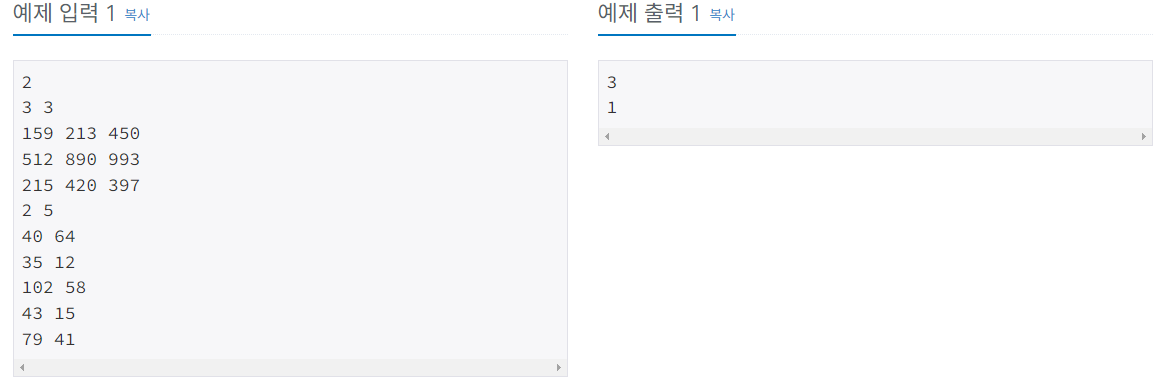
통과한 답안
namespace _16175
{
internal class Program
{
static void Main(string[] args)
{
int T = int.Parse(Console.ReadLine());
for (int test = 0; test < T; test++)
{
string[] inputs = Console.ReadLine().Split(' ');
int N = int.Parse(inputs[0]);
int M = int.Parse(inputs[1]);
int[] candidates = new int[N];
for (int i = 0; i < M; i++)
{
string[] votes = Console.ReadLine().Split(' ');
for (int j = 0; j < N; j++)
{
candidates[j] += int.Parse(votes[j]);
}
}
int maxIdx = candidates.Select((value, index) => new { value, index })
.OrderByDescending(x => x.value)
.First()
.index;
Console.WriteLine(maxIdx + 1);
}
}
}
}
T개의 테스트 수가 주어지고, 각각의 테스트마다 N명의 지원자, M개의 지역이 주어진다.
그 후에 M개의 지역에서 각각 지원자들에게 투표된 표 수가 주어진 경우
가장 많은 표를 받은 지원자를 찾는 문제이다.
입력값을 입력 받는 과정에서 int 배열을 이용하여 각각의 지원자들이 받은 표 수를 더하고
이 배열을 이용하여 가장 많은 표를 받은 지원자의 인덱스를 찾는 방식으로 구현하였다.
LINQ를 이용하여 값과 인덱스 쌍을 만들고, 이를 내림차순으로 정렬하고
첫 번째로 나오는 값의 인덱스를 구하도록 구현하였다.
'IT > BaekJoon' 카테고리의 다른 글
[BAEKJOON] 백준 1544: 사이클 단어 (C#) (0) | 2024.08.16 |
---|---|
[BAEKJOON] 백준 4402: Soundex (C#) (0) | 2024.08.16 |
[BAEKJOON] 백준 5338: 마이크로소프트 로고 (C#) (1) | 2024.08.15 |
[BAEKJOON] 백준 11816: 8진수, 10진수, 16진수 (C#) (0) | 2024.08.13 |
[BAEKJOON] 백준 9070: 장보기 (C#) (0) | 2024.08.13 |